Introduction
I will show you how to backup site or application code and database in PHP based Codeigniter framework. In the world of web it is important to take backup of our site and database frequently. You don’t know when you’d come across unexpected situation which causes your site stop working. Here I have developed an application in Codeigniter, which will help you to take backup the application code and database.
While most web hosting company do a daily backup of a customer’s database and site, relying on them to make backups and provide them at no cost is risky also.
If you do not want it to use with Codeigniter you could also use it in simple PHP application and for that you just need to understand the logic carefully so that you can convert the code into equivalent PHP.
Prerequisites
Apache 2.4 http server, PHP 7.4.3, Codeigniter 3.1.11, MySQL 8.0.17
Note I have used
md5()
for encrypting some values. It’s up to you which algorithm you want to use.
Please go through the below sections for coding example on how to take backup site and database using Codeigniter.
Project Directory
It’s assumed that you have setup Apache, PHP and Codeigniter in Windows system.
Now I will create a project root directory called codeIgniter-backup-site-and-db the Apache server’s htdocs folder.
Now move all the directories and files from CodeIgniter framework into codeIgniter-backup-site-and-db directory.
I may not mention the project root directory in subsequent sections and I will assume that I am talking with respect to the project root directory.
MySQL Tables
First thing is you need to create database tables. The below backup table stores the information for backup.
CREATE TABLE IF NOT EXISTS `backup` (
`backup_id` int unsigned COLLATE utf8mb4_unicode_ci NOT NULL AUTO_INCREMENT,
`backup_name` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
`backup_location` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
`created_date` timestamp COLLATE utf8mb4_unicode_ci NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`backup_id`),
UNIQUE KEY `backup_name_UNIQUE` (`backup_name`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
The below session table stores session information for each client or user who access this backup page. This session table is used only if you are going to store session information into table. For this example I am saving session information into file.
CREATE TABLE IF NOT EXISTS `ci_sessions` (
`id` varchar(128) COLLATE utf8mb4_unicode_ci NOT NULL,
`ip_address` varchar(45) COLLATE utf8mb4_unicode_ci NOT NULL,
`timestamp` int(10) unsigned COLLATE utf8mb4_unicode_ci DEFAULT 0 NOT NULL,
`data` blob NOT NULL,
KEY `ci_sessions_timestamp` (`timestamp`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
If you do not want to save the session information then you can use default session storage mechanism – file system. To use default session storage mechanism you just need to specify the physical path for sess_save_path in application/config/config.php file. For example, if you want to save into application/cache directory then set as sess_save_path = APPPATH . 'cache/';
Directory – assets
Create assets directory, where css, images and js files will reside, at the root level i.e. parallel to application directory as shown into the picture.
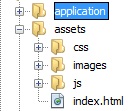
Download images from the below link and put into assets/images directory
Directory – backups
Create backups/databases and backups/sites directory which will hold the backups for databases and sites correspondingly as shown below picture:
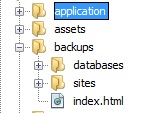
Database Configurations
Configure database settings at location application/config/database.php.
...
'hostname' => 'localhost',
'username' => 'root',
'password' => 'root',
'database' => 'roytuts',
...
'char_set' => 'utf8mb4',
'dbcollat' => 'utf8mb4_general_ci',
...
Session Configuration
Modify the config.php
file at location application/config/config.php for session.
...
$config['encryption_key'] = '2d8+e6]K0?ocWp7&`K)>6Ky"|.x|%nuwafC~S/+6_mZI9/17y=sKKG.;Tt}k';
...
$config['sess_driver'] = 'files';
$config['sess_cookie_name'] = 'ci_session';
$config['sess_expiration'] = 7200;
$config['sess_save_path'] = APPPATH . 'cache/';
$config['sess_match_ip'] = FALSE;
$config['sess_time_to_update'] = 300;
$config['sess_regenerate_destroy'] = FALSE;
...
Autoload Configuration
Modify the autoload.php file at location application/config/ to auto-load few drivers and helpers to avoid repeated loading such things.
$autoload['libraries'] = array('database', 'session');
$autoload['helper'] = array('url', 'file', 'text', 'form');
View File
This view file displays the page on UI (User Interface) and user will select the appropriate input to take backups. The following code is written into application/views/backup.php file.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
.h_title{
background: url(assets/images/bg_box_head.jpg) repeat-x;
color: #bebebe;
cursor: pointer;
font-size: 14px;
font-weight: normal;
height: 22px;
padding: 7px 0 0 15px;
text-shadow: #0E0E0E 0px 1px 1px;
}
.message_box {
margin-top: 10px;
}
.error, .success {
/*padding: 20px 20px 20px 40px;*/
max-width: 525px;
margin: auto;
padding: 10px 10px 10px 45px;
margin-bottom: 5px;
border-style: solid;
border-width: 1px;
background-position: 10px 10px;
background-repeat: no-repeat;
}
.error {
background-color: #f5dfdf;
background-image: url(assets/images/error.png);
border-color: #ce9e9e;
}
.success {
background-color: #e8f5df;
background-image: url(assets/images/success.png);
border-color: #9ece9e;
}
</style>
</head>
<body>
<div class="h_title">
Codeigniter Site and Database Backup
</div>
<div class="message_box">
<?php
if (isset($success) && strlen($success)) {
echo '<div class="success">';
echo '<p>' . $success . '</p>';
echo '</div>';
}
if (isset($errors) && strlen($errors)) {
echo '<div class="error">';
echo '<p>' . $errors . '</p>';
echo '</div>';
}
if (validation_errors()) {
echo validation_errors('<div class="error">', '</div>');
}
?>
</div>
<?php
$back_url = $this->uri->uri_string();
$key = 'referrer_url_key';
$this->session->set_flashdata($key, $back_url);
?>
<div class="body body-s">
<?php
echo form_open($this->uri->uri_string());
?>
<fieldset>
<section>
<label>Backup Type</label>
<label>
<select name="backup_type">
<option value="" selected disabled>Backup Type</option>
<option value="1" <?php echo (isset($success) && strlen($success) ? '' : (set_value('backup_type') == '1' ? 'selected' : '')) ?>>DB Backup</option>
<option value="2" <?php echo (isset($success) && strlen($success) ? '' : (set_value('backup_type') == '2' ? 'selected' : '')) ?>>Site Backup</option>
</select>
</label>
</section>
<section>
<label>File Type</label>
<label>
<select name="file_type">
<option value="" selected disabled>File Type</option>
<option value="1" <?php echo (isset($success) && strlen($success) ? '' : (set_value('file_type') == 1 ? 'selected' : '')) ?>>ZIP</option>
<option value="2" <?php echo (isset($success) && strlen($success) ? '' : (set_value('file_type') == 2 ? 'selected' : '')) ?>>GZIP</option>
</select>
</label>
</section>
</fieldset>
<footer>
<button type="submit" name="backup" value="backup" class="button">Get Backup</button>
</footer>
<?php
echo form_close();
?>
</div>
</body>
</html>
Model Class
This model is responsible for saving backup to databse and deleting backup from database table. The following code is written into application/models/Backup_Model.php file.
<?php
if (!defined('BASEPATH'))
exit('No direct script access allowed');
/**
* Description of backup_model
*
* @author https://roytuts.com
*/
class Backup_model extends CI_Model {
private $backup = 'backup';
/**
* save backup details
*
* @return boolean
*/
function save_backup_details($file_name, $file_path) {
$this->db->trans_start();
$data = array(
'backup_name' => $file_name,
'backup_location' => $file_path,
'created_date' => date('Y-m-d H:i:s')
);
$this->db->insert($this->backup, $data);
$id = $this->db->insert_id();
$this->db->trans_complete();
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
return NULL;
} else {
$this->db->trans_commit();
return $id;
}
return NULL;
}
/**
* delete db backup file
*
* @return boolean
*/
function delete_db_file($file_id) {
$this->db->trans_start();
$this->db->where('backup_id', $file_id);
$query = $this->db->get($this->backup);
$temp = $query->row();
$this->db->where('backup_id', $file_id);
$this->db->delete($this->backup);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
return NULL;
} else {
$this->db->trans_commit();
return $temp;
}
}
/**
* check file exists in db
*
* @return boolean
*/
function check_db_file($file_id) {
$this->db->where('backup_id', $file_id);
$this->db->limit(1);
$query = $this->db->get($this->backup);
if ($query->num_rows() == 1) {
return $query->row();
}
return NULL;
}
/**
* check file exists in db
*
* @return boolean
*/
function check_site_file($file_id) {
$this->db->where('backup_id', $file_id);
$this->db->limit(1);
$query = $this->db->get($this->backup);
if ($query->num_rows() == 1) {
return $query->row();
} else {
return NULL;
}
}
/**
* delete site backup file
*
* @return boolean
*/
function delete_site_file($file_id) {
$this->db->trans_start();
$this->db->where('backup_id', $file_id);
$query = $this->db->get($this->backup);
$temp = $query->row();
$this->db->where('backup_id', $file_id);
$this->db->delete($this->backup);
if ($this->db->trans_status() === FALSE) {
$this->db->trans_rollback();
return NULL;
} else {
$this->db->trans_commit();
return $temp;
}
}
}
/* End of file backup_model.php */
/* Location: ./application/models/Backup_Model.php */
Controller Class
This controller does everything for you like taking backup, downloading backup, deleting backup, saving backup to database, saving backup to disk space etc. The following code is written into application/controllers/Backup.php file.
<?php
if (!defined('BASEPATH'))
exit('No direct script access allowed');
/**
*
* @author https://roytuts.com
*/
class Backup extends CI_Controller {
/**
* error messages
*/
private $error;
/**
* success messages
*/
private $success;
/**
* base path or root directory of the site i.e. <drive>:/<folder>/<folder>/ or /<folder>/<folder>/
*/
public $base_path = "";
/**
* folder names to backup that are in the same directory as the CI index.php
* these can either be just 1 folder name "application" or a path like "application/controllers"
* if this array is empty then entire site structure is backed up
* public $directories = array("application", "forum", "assets/images", "assets/js", "assets/css", "system");
*/
private $directories = array();
/**
* containts a list of all the directories to ignore, leave empty to backup all
*/
private $ignore_directories = array('backups');
/**
* the directory name used for the temp file copy when everything is backed up
*/
private $copy_directory = "site_backup";
/**
* used to mark that the directory structure has alread been copied
*/
private $structure_copied = FALSE;
/**
* backup home url, i.e., http://www.example.com/backup
* access backup home page where you can create site and database backup
*/
private $home_url = 'backup';
/**
* site backup download url, i.e., http://www.example.com/download_site_file
* clicking on this url the site backup will be downloaded
*/
private $site_download_url = 'backup/download_site_file/';
/**
* site backup delete url, i.e., http://www.example.com/delete_site_file
* clicking on this url the site backup will be deleted
*/
private $site_delete_url = 'backup/delete_site_file/';
/**
* db backup download url, i.e., http://www.example.com/download_db_file
* clicking on this link the database backup will be downloaded
*/
private $db_download_url = 'backup/download_db_file/';
/**
* db backup delete url, i.e., http://www.example.com/delete_db_file
* clicking on this url the database backup will be deleted
*/
private $db_delete_url = 'backup/delete_db_file/';
/**
* this referrer_url_key is used to store the referrer url
* this referrer url will be used for backup operations like backup, download, delete
* after backup operation is over then we want to redirect to referrer url
*/
private $back_url_key = 'referrer_url_key';
private $back_url = 'backup';
private $db_backup_path = 'backups/databases/';
private $site_backup_path = 'backups/sites/';
function __construct() {
parent::__construct();
$this->load->dbutil();
$this->load->library('zip');
$this->load->library('form_validation');
$this->load->model('backup_model', 'backup');
$this->back_url = $this->session->flashdata($this->back_url_key);
}
private function handle_error($err) {
$this->error .= $err . "\r\n"; // "\r\n" means each error message will display in a new line
}
private function handle_success($succ) {
$this->success .= $succ . "\r\n"; // "\r\n" means each error message will display in a new line
}
/**
* display the main backup view page
*/
function index() {
if ($this->input->post('backup')) {
$this->form_validation->set_rules('backup_type', 'Backup Type', 'required');
$this->form_validation->set_rules('file_type', 'File Type', 'required');
if ($this->form_validation->run($this)) {
$backup_type = $this->input->post('backup_type');
$file_format = $this->input->post('file_type');
if (trim($backup_type) == 1) {
$this->get_db_backup($this->db_backup_path, $backup_type, $file_format);
} else if (trim($backup_type) == 2) {
$this->get_site_backup($this->site_backup_path, $backup_type, $file_format);
}
}
}
$data['errors'] = $this->error;
$data['success'] = $this->success;
$this->load->view('backup', $data);
}
/**
*
* db_backup
*
* this backs up database
*
* @access private
*/
private function get_db_backup($file_path, $backup_type, $file_format = 1) {
$this->load->helper('string');
$key_name1 = md5(date("d_m_Y_H_i_s")) . '_';
$key_name2 = '_db';
$key_name3 = date("d_m_Y_H_i_s");
if ($file_format == 1) {
//strong file name
$file_name = $key_name1 . $key_name3 . $key_name2 . '.zip';
$prefs = array(
'ignore' => array($this->ignore_directories),
'format' => 'zip', // gzip, zip, txt
'filename' => $file_name, // File name - NEEDED ONLY WITH ZIP FILES
'add_drop' => TRUE, // Whether to add DROP TABLE statements to backup file
'add_insert' => TRUE, // Whether to add INSERT data to backup file
'newline' => "\n" // Newline character used in backup
);
//Backup your entire database and assign it to a variable
//$backup = & $this->dbutil->backup($prefs);
$backup = $this->dbutil->backup($prefs);
$file = $file_path . $file_name;
if (strlen($backup)) {
if (!write_file($file, $backup)) {
$this->handle_error('Error while writing db backup to disk: ' . $file_name);
} else {
$this->handle_success('File Name: ' . $file_name . '. ');
$this->handle_success('DB backup successfully written to disk. ');
$date_arr = explode('_', $key_name3);
$date = $date_arr[0] . '-' . $date_arr[1] . '-' . $date_arr[2] . ' ' . $date_arr[3] . ':' . $date_arr[4] . ':' . $date_arr[5];
$saved_data = $this->backup->save_backup_details($file_name, $file_path);
if ($saved_data !== NULL) {
$this->handle_success('DB Backup details successfully saved to database. ');
$this->handle_success('You can download db backup here ' . anchor($this->db_download_url . $saved_data, 'download', array('class' => 'download')));
$this->handle_success('You can delete db backup here ' . anchor($this->db_delete_url . $saved_data, 'delete', array('class' => 'delete', 'onclick' => "return confirm('Are you sure want to delete this file ?')")));
} else {
if (file_exists($file)) {
unlink($file);
}
$this->handle_error('Error while saving db backup to database: ' . $file_name);
}
}
} else {
$this->handle_error('Error while getting db backup: ' . $file_name);
}
} else if ($file_format == 2) {
$file_name = $key_name1 . $key_name3 . $key_name2 . '.sql.gz';
$prefs = array(
'ignore' => array($this->ignore_directories),
'format' => 'gzip', // gzip, zip, txt
'filename' => $file_name, // File name - NEEDED ONLY WITH ZIP FILES
'add_drop' => TRUE, // Whether to add DROP TABLE statements to backup file
'add_insert' => TRUE, // Whether to add INSERT data to backup file
'newline' => "\n" // Newline character used in backup file
);
//Backup your entire database and assign it to a variable
$backup = & $this->dbutil->backup($prefs);
$file = $file_path . $file_name;
if (strlen($backup)) {
if (!write_file($file, $backup)) {
$this->handle_error('Error while writing db backup to disk: ' . $file_name);
} else {
$this->handle_success('File Name: ' . $file_name . '. ');
$this->handle_success('DB backup successfully written to disk. ');
$date_arr = explode('_', $key_name3);
$date = $date_arr[0] . '-' . $date_arr[1] . '-' . $date_arr[2] . ' ' . $date_arr[3] . ':' . $date_arr[4] . ':' . $date_arr[5];
$saved_data = $this->backup->save_backup_details($file_name, $file_path);
if ($saved_data !== NULL) {
$this->handle_success('DB Backup details successfully saved to database. ');
$this->handle_success('You can download db backup here ' . anchor($this->db_download_url . $saved_data, 'download', array('class' => 'download')));
$this->handle_success('You can delete db backup here ' . anchor($this->db_delete_url . $saved_data, 'delete', array('class' => 'delete', 'onclick' => "return confirm('Are you sure want to delete this file ?')")));
} else {
if (file_exists($file)) {
unlink($file);
}
$this->handle_error('Error while saving db backup to database: ' . $file_name);
}
}
} else {
$this->handle_error('Error while getting db backup: ' . $file_name);
}
}
}
/**
*
* delete db file
*
* delete backup of database
*
* @access public
*/
function delete_db_file($file_id) {
$this->session->keep_flashdata($this->back_url_key);
if (strlen(trim($this->back_url)) <= 0 || $this->back_url == NULL) {
$this->back_url = $this->home_url;
}
$file_data = $this->backup->delete_db_file($file_id);
if ($file_data !== NULL) {
$file = $file_data->backup_location . $file_data->backup_name;
if (file_exists($file)) {
unlink($file);
}
}
redirect($this->back_url);
}
/*
*
* delete db file
*
* delete backup of the entire site
*
* @access public
*/
function download_db_file($file_id) {
$this->session->keep_flashdata($this->back_url_key);
if (strlen(trim($this->back_url)) <= 0 || $this->back_url == NULL) {
$this->back_url = $this->home_url;
}
$file_data = $this->backup->check_db_file($file_id);
if ($file_data !== NULL) {
$this->load->helper('download');
$file_path = $this->db_backup_path . $file_data->backup_name;
$data = file_get_contents($file_path); // Read the file's contents
$filename = basename($file_path);
$ext = pathinfo($filename, PATHINFO_EXTENSION);
$name = md5(date('d-m-Y H:i:s')) . date('_d-m-Y_H:i:s') . '.' . $ext;
force_download($name, $data);
}
redirect($this->back_url);
}
/**
*
* delete_site_file
*
* delete backup of the database
*
* @access public
*/
function delete_site_file($file_id) {
$this->session->keep_flashdata($this->back_url_key);
if (strlen(trim($this->back_url)) <= 0 || $this->back_url == NULL) {
$this->back_url = $this->home_url;
}
$file_data = $this->backup->delete_site_file($file_id);
if ($file_data !== NULL) {
$file = $file_data->backup_location . $file_data->backup_name;
if (file_exists($file)) {
unlink($file);
}
}
redirect($this->back_url);
}
/*
*
* download_site_file
*
* download the file after successful backup of the entire site
*
* @access public
*/
function download_site_file($file_id) {
$this->session->keep_flashdata($this->back_url_key);
if (strlen(trim($this->back_url)) <= 0 || $this->back_url == NULL) {
$this->back_url = $this->home_url;
}
$file_data = $this->backup->check_site_file($file_id);
if ($file_data !== NULL) {
$this->load->helper('download');
$file_path = $file_data->backup_location . $file_data->backup_name;
$data = file_get_contents($file_path); // Read the file's contents
$filename = basename($file_path);
$ext = pathinfo($filename, PATHINFO_EXTENSION);
$name = md5(date('d-m-Y H:i:s')) . date('_d-m-Y_H:i:s') . '.' . $ext;
force_download($name, $data);
}
redirect($this->back_url);
}
/**
*
* site_backup
*
* this backs up all the files located in the directories
* specified.
*
* @access private
*/
private function get_site_backup($file_path, $backup_type, $file_format = 1) {
$this->zip->clear_data();
$this->check_directory($file_path);
$this->check_directory($this->copy_directory);
//loop each of the folder that will be backed up
if (count($this->directories) > 0) {
foreach ($this->directories as $dir) {
if (!in_array($dir, $this->ignore_directories)) {
$location = $this->base_path . $dir . "/";
$this->zip->read_dir($location, FALSE);
}
}
} else {
//takes a copy of the code to ensure that the backup of backups is not made.
$copied = FALSE;
if ($this->structure_copied === FALSE) {
$path = str_replace('\\', '/', realpath($this->base_path));
$copied = $this->copy_site_files($path, $this->base_path . $this->copy_directory . "/");
}
if (($copied === TRUE) || ($this->structure_copied === TRUE)) {
$this->zip->read_dir($this->base_path . $this->copy_directory . "/", FALSE);
}
}
flush();
$key_name = date("d_m_Y_H_i_s");
if ($file_format == 1) {
$file_name = md5($key_name) . '_site.zip';
$zipped = $this->zip->archive($file_path . $file_name);
$this->zip->clear_data();
if ($this->structure_copied === TRUE) { //we need to remove the copied files to ensure that the server is kept nice and tidy
$this->remove_temp_files($this->base_path . $this->copy_directory . "/");
}
if ($zipped == 1) {
$this->handle_success('File Name: ' . $file_name . '. ');
$this->handle_success('Site backup successfully written to disk. ');
$date_arr = explode('_', $key_name);
$date = $date_arr[0] . '-' . $date_arr[1] . '-' . $date_arr[2] . ' ' . $date_arr[3] . ':' . $date_arr[4] . ':' . $date_arr[5];
$file = $file_path . $file_name;
$saved_data = $this->backup->save_backup_details($file_name, $file_path, $backup_type);
if ($saved_data !== NULL) {
$this->handle_success('Site Backup details successfully saved to database. ');
$this->handle_success('You can get site backup here ' . anchor($this->site_download_url . $saved_data, 'download', array('class' => 'download')));
$this->handle_success('You can delete site backup here ' . anchor($this->site_delete_url . $saved_data, 'delete', array('class' => 'delete', 'onclick' => "return confirm('Are you sure want to delete this file ?')")));
} else {
if (file_exists($file)) {
unlink($file);
}
$this->handle_success('Error while saving site backup to database: ' . $file_name);
}
} else {
$this->handle_error('Error while writing site backup to disk: ' . $file_name);
}
} else if ($file_format == 2) {
$file_name = md5($key_name) . '_site.tar.gz';
$zipped = $this->zip->archive($file_path . $file_name);
$this->zip->clear_data();
if ($this->structure_copied === TRUE) { //we need to remove the copied files to ensure that the server is kept nice and tidy
$this->remove_temp_files($this->base_path . $this->copy_directory . "/");
}
if ($zipped == 1) {
$this->handle_success('File Name: ' . $file_name . '. ');
$this->handle_success('Site backup successfully written to disk. ');
$date_arr = explode('_', $key_name);
$date = $date_arr[0] . '-' . $date_arr[1] . '-' . $date_arr[2] . ' ' . $date_arr[3] . ':' . $date_arr[4] . ':' . $date_arr[5];
$file = $file_path . $file_name;
$saved_data = $this->backup->save_backup_details($file_name, $file_path, $backup_type);
if ($saved_data !== NULL) {
$this->handle_success('Site Backup details successfully saved to database. ');
$this->handle_success('You can get site backup here ' . anchor($this->site_download_url . $saved_data, 'download', array('class' => 'download')));
$this->handle_success('You can delete site backup here ' . anchor($this->site_delete_url . $saved_data, 'delete', array('class' => 'delete', 'onclick' => "return confirm('Are you sure want to delete this file ?')")));
} else {
if (file_exists($file)) {
unlink($file);
}
$this->handle_success('Error while saving site backup to database: ' . $file_name);
}
} else {
$this->handle_error('Error while writing site backup to disk: ' . $file_name);
}
}
}
/**
*
* copy_site_files
*
* this copies all the files located in the site
* specified.
*
* @access private
*/
private function copy_site_files($path, $dest) {
if (is_dir($path)) {
@mkdir($dest);
$objects = scandir($path);
if (sizeof($objects) > 0) {
foreach ($objects as $file) {
if ($file == "." || $file == "..") {
continue;
}
// go on
if (is_dir($path . "/" . $file)) {
if ((!in_array($file, $this->ignore_directories)) && ($file != $this->copy_directory)) {
$this->copy_site_files($path . "/" . $file, $dest . "/" . $file);
}
} else {
copy($path . "/" . $file, $dest . "/" . $file);
}
}
}
$this->structure_copied = TRUE;
return TRUE;
} elseif (is_file($path)) {
$this->structure_copied = TRUE;
return copy($path, $dest);
} else {
$this->structure_copied = TRUE;
return FALSE;
}
}
/*
*
* remove_temp_files
*
* this removes all the files and directories located in the directory
*
* WRITTEN BY: O S 18-Jun-2010 10:30 from the PHP manual rmdir comments
*
* WANRING: if you run this on you base_path then all the files in the site will be deleted
*
* @access private
*/
private function remove_temp_files($directory) {
if (substr($directory, -1) == "/") {
$directory = substr($directory, 0, -1);
}
if (!file_exists($directory) || !is_dir($directory)) {
return FALSE;
} elseif (!is_readable($directory)) {
return FALSE;
} else {
$directoryHandle = opendir($directory);
while ($contents = readdir($directoryHandle)) {
if ($contents != '.' && $contents != '..') {
$path = $directory . "/" . $contents;
if (is_dir($path)) {
$this->remove_temp_files($path);
} else {
unlink($path);
}
}
}
closedir($directoryHandle);
if (!rmdir($directory)) {
return FALSE;
} else {
return TRUE;
}
}
}
/*
*
* check_directory
*
* checks the directory to make sure it exists before we attempt to create the file
*
* @params string
* @access private
*/
private function check_directory($path) {
if (!@opendir($path)) {
mkdir($path, 0755);
} //if(!@opendir($path))
return;
}
}
/* End of file admin.php */
/* Location: ./application/controllers/Backup.php */
Testing the Application
Now run the application. Make sure that your web server and MySQL server are running.
Home Page
Hit the URL http://localhost/codeIgniter-backup-site-and-db in the browser.
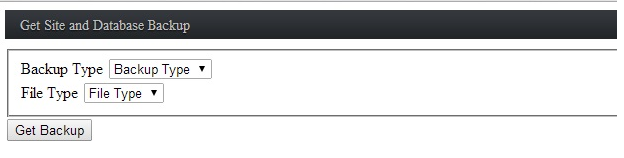
Error Message
If inputs are not selected.
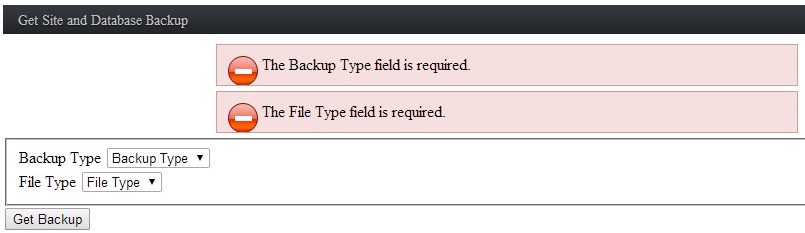
Backup – Get
When you select the inputs and click on Get Backup button. You can download or delete the backup using the links.
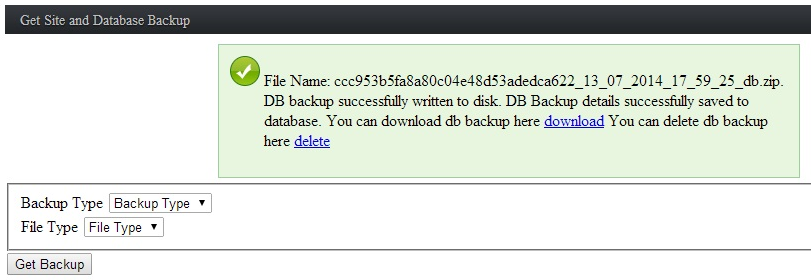
Thank you So much Sir for your code. Really Appreciated