This tutorial will show you how to create dependent or cascaded dynamic dropdown using jQuery. Sometimes you need to create such a cascaded dropdown where you need to select some value in the second dropdown based on first dropdown value. For example, you may need to select city dropdown value based on country dropdown value and for this you cannot pre-populate the city dropdown value unless you know the country value from country dropdown.
I will explain here with an example using jQuery. The example here contains two dropdown – one is parent dropdown that contains Continents & Oceans and another is sub-dropdown that contains continents’ names & oceans’ names and will be displayed based on selected parent dropdown value.
I am using jQuery from CDN (Content Delivery Network) and I have tested with jQuery versions 2 and 3.
Prerequisites
HTML, jQuery
Final Output
When home page opens you will see the following output:
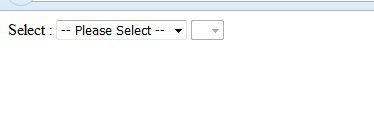
When parent select box is selected then you will see the following output:
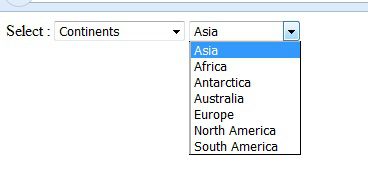
Dependent Dropdown
The following code shows how to implement dependent dropdown or cascaded dropdown using HTML and jQuery library.
<!DOCTYPE html>
<html>
<head>
<meta charset='UTF-8' />
<!--<script src="http://ajax.googleapis.com/ajax/libs/jquery/2.0.0/jquery.min.js"></script>-->
<script src="https://code.jquery.com/jquery-3.5.1.min.js" integrity="sha256-9/aliU8dGd2tb6OSsuzixeV4y/faTqgFtohetphbbj0=" crossorigin="anonymous"></script>
<script language="javascript" type="text/javascript">
$(document).ready(function() {
//create arrays to store option and values
var continents = [
{display: "Asia", value: "asia" },
{display: "Africa", value: "africa" },
{display: "Antarctica", value: "antarctica" },
{display: "Australia", value: "australia" },
{display: "Europe", value: "europe" },
{display: "North America", value: "north-america" },
{display: "South America", value: "south-america" }
];
var oceans = [
{display: "Pacific", value: "pacific" },
{display: "Atlantic", value: "atlantic" },
{display: "Indian", value: "indian" },
{display: "Southern", value: "southern" },
{display: "Arctic", value: "arctic" }
];
//If parent option is changed
$("#parent_selection").change(function() {
var parent = $(this).val(); //get option value from parent
switch(parent){ //using switch compare selected option and populate child
case 'continents':
$('#child_selection').attr('disabled', false);
list(continents);
break;
case 'oceans':
$('#child_selection').attr('disabled', false);
list(oceans);
break;
default: //default child option is blank
$("#child_selection").html('');
$('#child_selection').attr("disabled", true);
break;
}
});
//function to populate child select box
function list(array_list) {
$("#child_selection").html(""); //reset child options
$(array_list).each(function (i) { //populate child options
$("#child_selection").append("<option value='" + array_list[i].value + "'>" + array_list[i].display + "</option>");
});
}
});
</script>
</head>
<body>
<div class="wrapper">
Select :
<select name="parent_selection" id="parent_selection">
<option value="">-- Please Select --</option>
<option value="continents">Continents</option>
<option value="oceans">Oceans</option>
</select>
<select name="child_selection" id="child_selection" disabled="disabled"/>
</div>
</body>
</html>
In the above code I have defined two arrays with values which will be shown in the parent and child dropdown. Based on the selected parent dropdown value you will see the values in child dropdown.
By default child dropdown selection is disabled until you select the parent dropdown’s value. I have used jQuery’s each()
function to iterate through each element of the array and append()
function to append to the dropdown for building select option.
Similar Posts:
It doesn’tn work. The combobox parent selection works but the childs selection combo box only show a little box with little dropdown triangle. Not change select oceans or continents.
I try to add in line child selection but useless.
There was a quotation mismatch. I updated it. You can check it now.