I am going to show you how to convert video (MP4/AVI) clips to gif in Python programming language. Recently I was trying online to convert an avi format video file to gif and I found that there are some limitations: you cannot upload more than 50 or 100 MB file; you need to register or login to the web site to convert your video file. And my requirement was not fulfilled. So I wrote this example.
The source code which I am going to show you can be found in the documentation of usage examples. In this link it is shown how to read and write the data during conversion from video to gif file.
Prerequisites
Python 3.9.1, imageio, imageio-ffmpeg
Install Required Modules
You need the required modules to be installed before converting from video clip to gif format. Here I will show you both AVI and MP4 video format conversion to GIF format.
Install imageio using the command pip install imageio
in the command line tool.
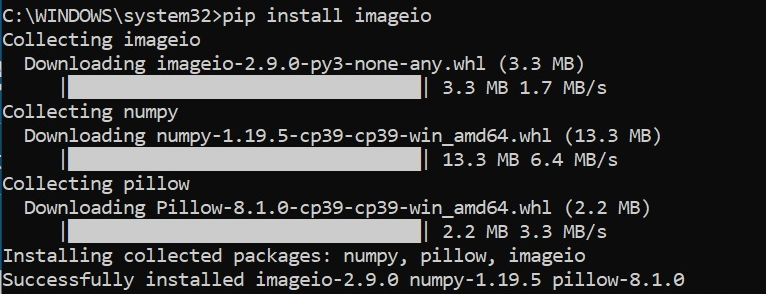
Install imageio-ffmpeg using the command pip install imageio-ffmpeg
in the command line tool.

Convert AVI/MP4 to GIF
Now I am going to write Python script to convert the desired video file into gif.
import imageio
import os
def convertVideoToGifFile(inputFile, outputFile=None):
if not outputFile:
outputFile = os.path.splitext(inputFile)[0] + ".gif"
print("Converting {0} to {1}".format(inputFile, outputFile))
reader = imageio.get_reader(inputFile)
fps = reader.get_meta_data()['fps']
writer = imageio.get_writer(outputFile, fps=fps)
for i,im in enumerate(reader):
writer.append_data(im)
writer.close()
print("\r\nConversion done.")
#Convert Input Files
convertVideoToGifFile("sample_960x540.avi")
convertVideoToGifFile("SampleVideo_1280x720_1mb.mp4", "SampleVideo.gif")
In the above Python code I have imported the required modules imageio and os.
I have defined a function convertVideoToGifFile()
to get the input video file and convert it to gif file. In this function I am checking if output file name with full path has been provided or not. If not provided then I am extracting the input file name which will be used as an output file name with .gif extension. Ideally you should perform all required validation for your input data.
next I am getting the reader and building the writer with the output file path. The input data get appended to the writer and finally the output file gets generated.
The quality of output depends on the input video. Sometimes the quality of the output gif may degrade.
Testing the Application
Simply executing the python script will generate the output gif files. In the above code I have put the input video files in the same directory as my Python script. The output gif files get generated in the same directory as input files.
