Introduction
The error, utility classes should not have a public or default constructor, you notice mainly in utility classes where you have declared your class method(s) as public static and your class does not have private constructor as well as your class cannot be made as final.
This error occurs due to the checkstyle rules defined generally in checkstyle.xml that is used to check the quality of the written Java code.
This not only occurs due to the use of checkstyle plugin but also due to the use of SonarQube or other static code quality checking tool for Java code.
Recommended reading: How to use checkstyle plugin in gradle based project
Problem Scenario
I was also working on gradle based Spring Boot application and when I executed the checkstyle plugin then I saw this error on the utility classes where I did not declare the class as final but I had private constructor in each class. So it was very easy to fix the problem in those utility classes. So I just made sure that those utility classes must have private constructor and the utility classes should be declared as final to remove such issue.
But when I executed the checkstyle rules on Spring Boot main class I also found the same issue. Now neither I can declare the @SpringBootApplication
main class final nor I can have a private constructor in Spring Boot main class because it is not a utility class.
If you make this Spring Boot class final and if you declare any private constructor in this main class, Spring will complain and won’t be able to run your application. Therefore I cannot use the same solution for this Spring Boot main class.
So I suppressed this issue for this main class by applying a rule in the suppressions.xml file that will be included into checkstyle.xml file in order to make it work.
The class which I am using in this example is givev below. You can download the whole source later from the source code section at the bottom of the tutorial.
package com.roytuts.checkstyle;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
*
* @author Soumitra
*
*/
@SpringBootApplication
public class SpringCheckStyleApp {
/**
*
* @param args
*/
public static void main(final String[] args) {
SpringApplication.run(SpringCheckStyleApp.class, args);
}
}
Prerequisites
Java at least 1.8, Spring Boot 2.3.3, Gradle 6.5.1
How to use checkstyle on gradle based Java project
suppressions.xml
Create supressions.xml file in the same directory (<projet’s root directory>/config/checkstyle) as you have put your checkstyle.xml file.
Put below content into supressions.xml file.
<?xml version="1.0"?>
<!DOCTYPE suppressions PUBLIC
"-//Puppy Crawl//DTD Suppressions 1.0//EN"
"https://checkstyle.org/dtds/suppressions_1_0.dtd">
<suppressions>
<suppress files="SpringCheckStyleApp.java" checks="HideUtilityClassConstructor" />
</suppressions>
Include the supressions.xml file into checkstyle.xml file by using the below code. The variable ${config_loc}
‘s value is resolved when you use the default location for checkstyle plugin. If you use location other than default then make sure the file is included.
You need to put the below content inside checkstyle.xml file before the last end tag of </module>
.
<module name="SuppressionFilter">
<property name="file" value="${config_loc}/suppressions.xml" />
</module>
Executing Chekstyle
Now execute the checkstyle rules, you should not see the issue – utility classes should not have a public or default constructor.
That’s all. Hope you got idea how to remove checkstyle issues from utility classes as well from @SpringBootApplication
main class.
Recommended reading: How to use checkstyle plugin in gradle based project
If you do not have any JavaDoc and package-info.java file then also you will get errors, so I have not discussed about them because other errors are already taken care in this example source code.
The errors you will receive generally as shown in the below image:
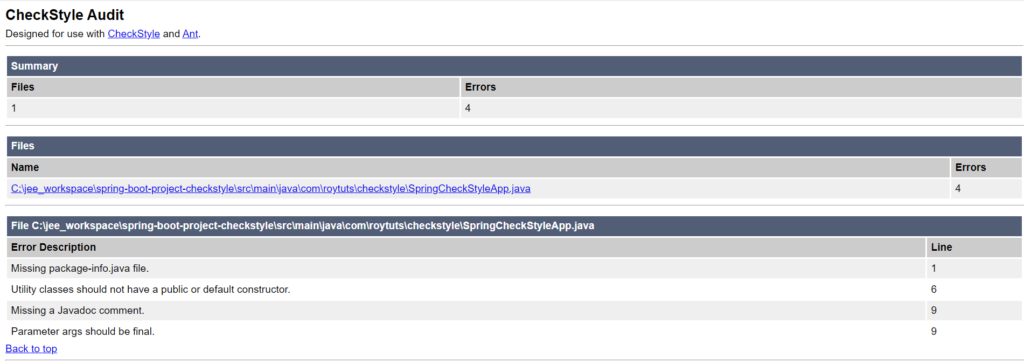
Source Code
Thanks for reading.