Introduction
In this guide I will tell you how to create header and footer in word document using Python 3. We will finally create docx file. Sometime you want to write some text in header and footer of word file to enhance the readability of viewers. Headers and footers are linked to a section; this allows each section to have a distinct header and/or footer.
A page header is text that appears in the top margin area of each page, separated from the main body of text, and usually conveying context information, such as the document title, author, creation date, or the page number. A page footer is analogous in every way to a page header except that it appears at the bottom of a page.
Related Posts:
Prerequisites
Python 3.8.0 – 3.9.1, Microsoft Office Word, docx 0.8.10 (pip install python-docx
)
Installing Module
Make sure you have the required module docx installed in your operating system.
You can check whether your module is exists or not in Python terminal by executing the command: import docx
. If module exists then you won’t get any error otherwise you would get error something like “module does not exist”.
Header and Footer in Word File
Next we will create Python script to create header and footer in word document.
from docx import Document
document = Document()
#header section
header_section = document.sections[0]
header = header_section.header
#footer section
footer_section = document.sections[0]
footer = footer_section.footer
#header text
header_text = header.paragraphs[0]
header_text.text = "Header of my document"
#heading of the document
document.add_heading('Document Title', 0)
#paragraph
p = document.add_paragraph('A plain paragraph')
#footer text
footer_text = footer.paragraphs[0]
footer_text.text = "Footer of my document"
#write to docx
document.save('simple.docx')
In the above Python script first I have imported the required package or module for word document.
Next I have created document object. Then I have extracted the header and footer sections.
Then I have set text to the header section followed by heading (document title) and a paragraph to the word document.
Next I have written text to footer.
Finally I have saved the word document as sample.docx.
Testing the Script
Running the above Python script will produce below word file:
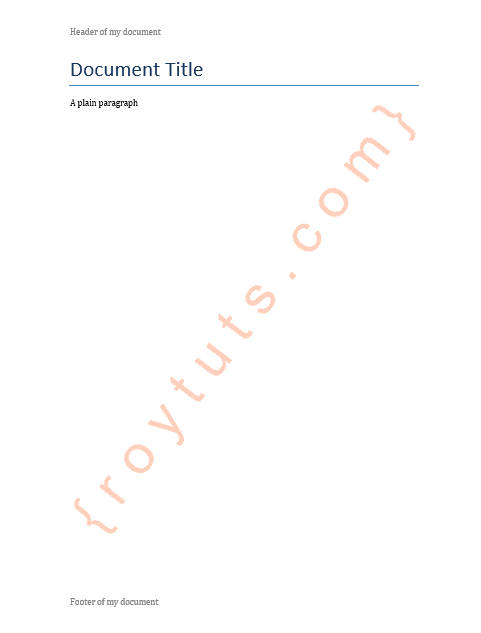
That’s all. Hope you got an idea on how to create header and footer in word document using Python.